|
3/04 |
4/04 |
<-Open Closed->
Last Free Code |
5/04 |
6/04 |
Most Recent
The game's development will be documented here, completely open source, with hopes that other mod
developers can use my code to build on and make their own. So all the code is free, provided as is,
and ready for anyone to copy. That said, however, if anyone tries to feed off or copy my mod idea
without my consent i will sue them in the face. Painfully. So you've been warned. It'd be nice to
give me credit if you use my code too. That's my 'legal' paragraph done...
Keep in mind that these first few entries aren't all that descriptive, mainly because I didn't start this
website until the 23rd.
13 March '04
- Coded basic game framework, custom gametype, HUD, pawn, player controller and mutator. This all from a tutorial
at The Unreal Wiki.
- Did some preliminary work on a gesture weapon, removed the assault rifle, shieldGun & translocator from
automatically being placed in player's inventory via the gametype mutator.
- *.int file was created and finished - the gametype registers properly now.
14 March '04
- Made 2 weapon firemode classes which were intended to drive the gesture system. Set them up correctly so that
primary fire does not work without secondary. The linkgun mesh was used as a placeholder.
- code cleanup.
15 March '04
- The weapon's primary firemode now spawns particle emitters, and makes a noise. I was going to use the
effectOffset property in the firemode to change where the particles spawn (as in, drawing the gesture).
- Did some fiddling with ultraEdit and set up a project properly. I used ctags to index all function definitions
from the ut2003 game for easy reference. There is a tutorial for this
here.
- Today I also discovered INTERACTIONS: they are great. I set up an interaction for the mouse cursor, which
should bring up the cursor regardless of which weapon is selected. Tutorial on doing this at
the wiki.
18 March '04
- The cursor is being rendered! I moved the code for gesturing to the player controller class for some reason,
though I can't remember why now.
- The weapon and its firemode classes are now only in place for visual effects.
- The interaction class references to the player controller now, via ViewportOwner.Actor
- The source code at this stage looks like this.
20 March '04
- Overloaded the updateRotation() function on the player controller so that when the cursor comes up the
screen stops rotating and responding to mouse movement. The basic framework for a gesture system is now complete.
- Caused the earlier particle effect (same as botSparks.u) to spawn at the cursor location properly.
- Interaction class commented properly and cleaned up.
- Annoying temporary biorifle sound removed from weapon primary fire.
- Primary and secondary fire classes and projectile class for the gesture weapon removed since all functionality
is now handled by the interaction class, and I dont need them anymore.
- Later on removed the temporary particle effect and finalised the gesture visuals to a pretty cool degree. These
take the form of an xEmitter which spawns in front of the player using screenToWorld() from the interaction
class, as well as some basic vector addition.
- The source code at this stage looks like this.
21 March '04
- Ported the whole thing to ut2004 which I bought 2 days ago. There were some small issues: the
updateRotation() in the playerController seemed to have changed and some other
minor things, but they weren't too bad.
- The temporary link gun weapon mesh was removed, since it looked dumb for screenshotting.
- Mutator code was added to remove all level pickup items since other weapons spawning was kinda dumb.
- Added some sounds to gesture mode- an ambient one which plays when in gesture mode, and a woosh
which plays when gesture mode is released. These are both set on the player's pawn.. the ambient one simply
is the pawn's ambient sound, and the other is called via font color="#009000">playSound().
Later on I will code it so that it makes a failure noise if the gesture is too sloppy to be recognised.
Removed the accessor functions on the player controller that werent necessary. I just put them in since I
am used to java. What a douche.
- The source code at this stage looks like this.
22 March '04
- I am having a lot of problems with erm, something. Everything works ok in singleplayer, but as soon as i test
it on a LAN game, the server is the only one who can draw gestures, and the clients can't even see the gesturing effect.
- I moved all code for spawning the gesture effect to the pawn class to try and replicate, but it still doesnt work.
23 March '04
- The gametype mutator now hides all the weapon pickup bases as well as removing all the level items.
- Got rid of the ugly temp hud. Now there is the power of.. NO HUD!
- Found some really nice functions that made the nightmare of vector maths seem less like a bad thing.
There is a fun little function in the player controller class called GetViewRotation(), which
returns a vector normal from the player's head, facing in the right direction. I simply had to multiply this
by the right number of unreal units to get the gesture effect to spawn away from the player. I now go:
spawnTo = grabinController(PlayerController(Controller))
.myGestureInt.ScreenToWorld(spawnTemp,playerCamLoc,)
+ (18 * vector(Controller.GetViewRotation()));
gEffect = spawn(Class'gestureProjEffect',self,,spawnTo,Rotation);
Why did i do this? So you can see the gesture drawing effect when you run forwards.
- Fixed effect ownership issue when spawned from pawn.. I didn't include the 'self' part in the spawn function before
since it eluded me somehow.
- Made this website, and this developer journal. I will try to update it at least weekly (:
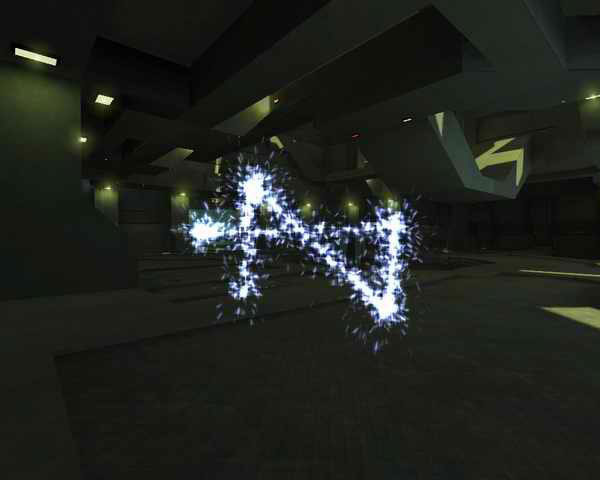 ^Final(?) gesture effect in action.
24 March '04
- Moved the effect a little closer to the player, since having it far away makes it difficult to see the correlation
between what you draw onscreen and what shows up. I set the effect xEmitter to have no particle delay too, so you
can pretty much always see it (a little bit) when you're walking forwards.
- The overloaded function updateRotation() in grabinController was dumb.
I killed off all the code and replaced it with a Super.updateRotation(). Whoops!
- Added some safeguards on referencing the player's pawn. Namely put IF statements with
if ( ViewportOwner.Actor.Pawn != None ) around those areas.
- The gesture interaction now checks the current weapon with
if ( ViewportOwner.Actor.Pawn.Weapon.isA('grabinGestureGun'))
before doing its thing. This way, i can add another weapon. But more on that later (:
25 March '04
- Realised the mutator code wasn't that great. Fiddled around with it. Nothing special though.
- Placed player pawn check code in other places to stop annoying warning messages in spectator mode. I should
make a note that every time you reference a player's pawn, you should check first if there actually IS one, using
if ( ViewportOwner.Actor.Pawn != None ).
- Set the interaction class so that view rotates in spectator... since not rotating was pretty annoying.
- Pawn class now lists the gesture gun as required equipment, not the mutator. This is probably better since the
mutator doesn't have to be running now for the weapon to spawn. Not that it ever wouldn't.
26 March '04
- Ive fiddled most of the code around a lot, moving it from one class to another to see if it has any effect. I've
isolated (at least part of) the problem with 'no gesture on LAN' - The pawn variables referencing the PlayerController and
its interaction return 'none'.. so that's probably a pretty major part of what's wrong.
27 March '04
- FIXED! - LAN game issues with non-spawning gesture particle effects and gesture interactions.
The problem was that the interaction didn't spawn on clients at ALL. Let's look at the old code in the PC (playerController):
auto state PlayerWaiting
{
Begin:
If ((Viewport(Player) != None) && (!bHasInteraction))
{
Player.InteractionMaster.AddInteraction("halfGrabin.gestureInter", Player);
bHasInteraction = True;
}
}
The problem with this, is that it only works pretty much once. If the player is in a preBeginPlay state as opposed
to a postBeginPlay.. in other words if they haven't ACTUALLY spawned yet.. then
Viewport(Player) will be none, and it will skip interaction assignment entirely, the
state will end, and nothing happens. At least, that's how i understand it.
To fix this, I instead put code in the mutator class, which checks until an assignment is made and the interaction
is added. The relevant code:
Class grabinMut extends Mutator config;
var bool bFindPlayer;
//Add interaction on CLIENTS as well as server
simulated function PostBeginPlay()
{
local Player P;
if (Level.NetMode != NM_DedicatedServer) //if not on dedicated (ie no player)
{
if (GetLocalPlayerController() == None) //hasn't spawned yet
{
bFindPlayer = True;
return;
}
P = GetLocalPlayerController().Player; // get the current player (not PC)
grabinController(P.Actor).myGestureInt =
P.InteractionMaster.AddInteraction("halfGrabin.gestureInter", P);
}
}
// saftey check to ensure we DID load the interaction client side
simulated function Tick(float DeltaTime)
{
local Player P;
if (bFindPlayer)
{
if (GetLocalPlayerController() == None) return;
P = GetLocalPlayerController().Player;
grabinController(P.Actor).myGestureInt =
P.InteractionMaster.AddInteraction("halfGrabin.gestureInter", P);
bFindPlayer = False;
Disable('Tick'); //can disable tick, interaction has been added
}
}
//iterator to return client's ACTUAL playerController
simulated function PlayerController GetLocalPlayerController()
{
local PlayerController PC;
if ( Level.NetMode == NM_DedicatedServer )
return None;
foreach DynamicActors(class'PlayerController', PC)
if ( Viewport(PC.Player) != None )
return PC;
return None;
}
......
defaultproperties
{
FriendlyName="halfGrabin Game Mutator"
Description="Grabin it in the banus"
bAlwaysRelevant=True
bOnlyDirtyReplication=False
RemoteRole=ROLE_SimulatedProxy
}
Here we see the bFindPlayer which checks if the interaction has been added. This is what happens:
First, we see if there is a PC yet in postBeginPlay(). If there is, the player
has spawned, and we can add the interaction and stop worrying. If not, they are still spectating, so we set
bFindPlayer to true. We now move down to the Tick().
We test to see if they have a PC yet (have spawned). If not, we return. No need to try the rest of the code.
If they have, we THEN call GetLocalPlayerController() to find out which PC
belongs to the player. Finally, we use that PC to reference the correct player and add the interaction in the
right place. myGestureInt is set on the PC so the pawn class has something to use
to access all the interaction based functions it needs.
It is also crucial that we set bAlwaysRelevant=True,
bOnlyDirtyReplication=False and RemoteRole=ROLE_SimulatedProxy
in the mutator's defaults, since the default settings for the base mutator class prevent it from running as a client
actor, and hence the Tick() will have no effect on clients without changing these
variables. At least, that's how I understand it. Correct me if i'm wrong by all means, but hey, this way works.
And yes, this does make all that replication code unnecessary for the moment.
- Added the same GetLocalPlayerController() function as above into the pawn class
to make that variable assignment work properly. It checks every 0.1 seconds, too, so if i ever incorporate
vehicles or any random shit like that, it'll all still work.
- Added if (PC != none) to the pawn's tick so that no annoying warning messages
pop up in spectator mode. Man, i hate the annoyances spectators bring about.
- Took away the mouse cursor drawing function in the interaction, since it is now kinda innacurate with the gesture
effect spawning so far in front of the player. I will simply add another spawned emitter later on to act as a cursor.
- Oh, and one more thing. I have only a few more assignments to do now, and most of tomorrow to do them in. I will
start coding, modelling and implementing a new melee weapon into the game very soon. Here's a look (a render, not a sketch. I
suck at sketches)-
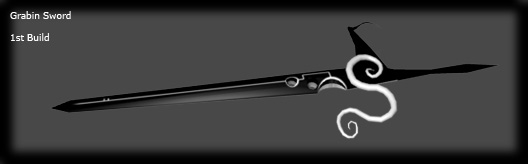
29 March '04
- There was a little problem in the pawn class... the overloaded GetLocalPlayerController()
has to actually be different here than in the mutator. Like this:
simulated function PlayerController GetLocalPlayerController()
{
local PlayerController PCon;
if ( Level.NetMode == NM_DedicatedServer )
return None;
foreach DynamicActors(class'PlayerController', PCon)
if ( PCon.Pawn == self )
return PCon;
return None;
}
As you can see, PCon.Pawn == Self is the test condition now. The other one actually
returns EVERY player controller in the level, which means that when the server draws they see everyone else
drawing too. It's kind of.. not good like that.
- You still can't see the xEmitter spawning from other players. damn. Also, you can't
hear other player's gesturing noise.
- Changed game name to 'Grabin' instead of 'half-grabin'.
- Added some replication statements and shifted some code around. I won't bother giving details until it fixes
this damn problem.
- Clients can see and hear server's gesture effects now, just not vice versa. I must be really close to fixing it
now, i guess. The whole problem which stopped the effect being seen was a simple
RemoteRole=ROLE_SimulatedProxy missing in the xEmitter class itself. I'm having a break
from gesturing though.. time to make a sword.
- Sword ingame, just fiddling around with ways to animate and make it look pretty, without actually creating any
new character animations. I will put a full write up about how i made the sword when it's done. Here's
some preliminary shots:
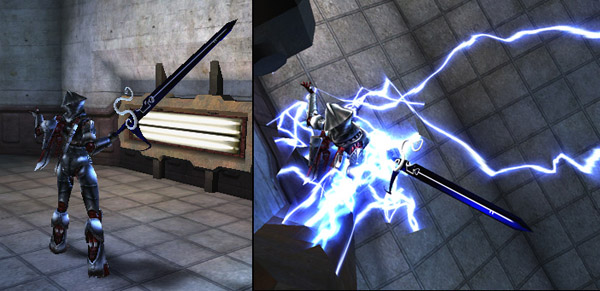
30 March '04
- Finally put the cursor back, this time so that all players can see it. It's another xEmitter, subclassing the
old one. It only shows when you're in gesture mode and not drawing, cos that looks cool. To make it work, i changed
the drawing() function in the pawn class, and made 2 new auxiliary functions, one of each
to be called in each state. The code looks like this:
function drawing()
{
playerCamLoc = Location + EyePosition();
//set location of player cam before function execution
spawnTemp.X = PC.Player.WindowsMouseX;
spawnTemp.Y = PC.Player.WindowsMouseY;
//vector to spawn effect to in relation to camera
spawnTo = PC.myGestureInt.ScreenToWorld(spawnTemp,playerCamLoc,) +
(5 * vector(GetViewRotation()));
}
function drawEffect()
{
//spawn effect at world center (effect should have warmup time)
gEffect = spawn(Class'gestureDrawFX',self,,spawnTo,);
//move effect to absolute coords
gEffect.Move(gEffect.Location + playerCamLoc);
}
function drawCursor()
{
hEffect = spawn(Class'gestureCursorFX',self,,spawnTo,);
hEffect.Move(hEffect.Location + playerCamLoc);
}
Easy stuff.
- I also changed the gesture effect to mimic the cursor effect, except for colour and lifetime. The cursor effect seriously
looked so much cooler than the gesture one. I don't know why, maybe I'm just accustomed to the gesture effect now.
The new effect is much more subtle, but it has some variation in the beam.
On the left is drawing effect, on the right is cursor effect:
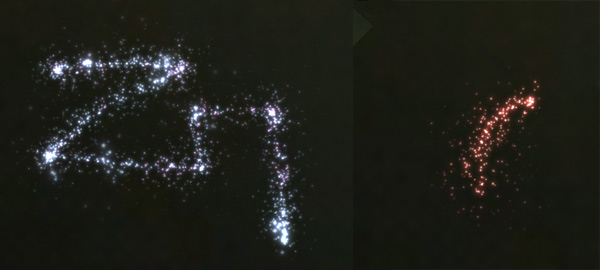
I did this with a real simple randomisation function in the pawn class:
function randomiseFX()
{
//this function just randomises the gesture particle FX a little in color and size
if (rand(10) == 1)
{
gEffect.mSizeRange[0]=2.000000;
gEffect.mSizeRange[1]=5.000000;
}
if (rand(4) == 1)
gEffect.skins[0]=Texture'EmitterTextures.Flares.EflareP2';
}
31 March '04
- And now i finally begin to fix this problem where the player can only see their own gestures. Firstly, I make
a new function in the pawn class to give itself an inventory:
function GiveGesture(string aClassName )
{
local class<Inventory> InvClass;
InvClass = class<Inventory>(DynamicLoadObject(aClassName, class'Class'));
if( FindInventoryType(InvClass) != None )
return;
GestureInv = Spawn(InvClass);
if( GestureInv != None )
GestureInv.GiveTo(self);
}
i declared GestureInv as a global variable. I then call this from the modifyPlayer() in the mutator like so:
Other.giveGesture("grabin.gestureInv");. This ensures that the gesture inventory is always
given to the player when they spawn. Now what I'm going to do is use this inventory class to completely handle all the
gesturing code. That means the effects as well as the checking and attack summons. I figure it's about time i started
sticking to some principles of OOP.
- Changed the gametype to do a few things related to player login and stuff. It's much easier to set up players
from here (;
3/04 |
4/04 |
<-Open Closed->
Last Free Code |
5/04 |
6/04 |
Most Recent
|
|